거두절미하고 바로 본론으로 들어가겠다.
우선 https://aws.amazon.com/ko/s3/에 접속해 계정을 생선한다.
계정 생성 후. 버킷 생성에 들어가
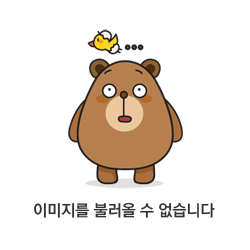
이름과 지역을 선택한다.
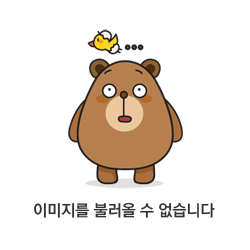
쭉 내려가다가
"이 버킷의 퍼블릭 엑세스 차단 설정"에서 모든 체크를 해제하고 만들기를 클릭한다.
이제 버킷이 만들어졌으면,
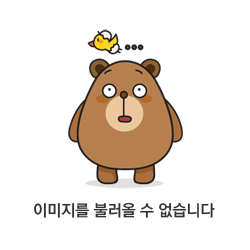
버킷을 하나 클릭한 후, 권한 > 버킷 정책에
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicListGet",
"Effect": "Allow",
"Principal": "*",
"Action": [
"s3:List*",
"s3:Get*"
],
"Resource": [
"arn:aws:s3:::Your_Bucket_Name",
"arn:aws:s3::Your_Bucket_Name/*"
]
}
]
}
을 복사해 붙인다.
Yout_Bucket_name에 자신의 버킷 이름을 적도록하자.
다시 같은 페이지에서 쭉 내리다보면 CORS가 나온다.
마찬가지로 여기에도
[
{
"AllowedHeaders": [
"*"
],
"AllowedMethods": [
"PUT",
"POST",
"DELETE",
"GET"
],
"AllowedOrigins": [
"*"
],
"ExposeHeaders": []
}
]
다음을 적어놓자.
이제 S3에서의 설정은 끝이났다.
VSCode로 돌아가서,
.env 파일과 S3Upload.js 파일을 만든다.
.env 파일에는
REACT_APP_REGION
REACT_APP_S3_BUCKET
를 각각 입력한다.
S3Upload.js에는
import React ,{useState} from 'react';
import AWS from 'aws-sdk'
const S3_BUCKET ='YOUR_BUCKET_NAME_HERE';
const REGION ='YOUR_DESIRED_REGION_HERE';
AWS.config.update({
accessKeyId: 'YOUR_ACCESS_KEY_HERE',
secretAccessKey: 'YOUR_SECRET_ACCESS_KEY_HERE'
})
const myBucket = new AWS.S3({
params: { Bucket: S3_BUCKET},
region: REGION,
})
const S3Upload = () => {
const [progress , setProgress] = useState(0);
const [selectedFile, setSelectedFile] = useState(null);
const handleFileInput = (e) => {
setSelectedFile(e.target.files[0]);
}
const uploadFile = (file) => {
const params = {
ACL: 'public-read',
Body: file,
Bucket: S3_BUCKET,
Key: file.name
};
myBucket.putObject(params)
.on('httpUploadProgress', (evt) => {
setProgress(Math.round((evt.loaded / evt.total) * 100))
})
.send((err) => {
if (err) console.log(err)
})
}
return <div>
<div>Native SDK File Upload Progress is {progress}%</div>
<input type="file" onChange={handleFileInput}/>
<button onClick={() => uploadFile(selectedFile)}> Upload to S3</button>
</div>
}
export default S3Upload;
를 적는다.
이제 컴포넌트를 삽입한 뒤, 이미지를 업로드 시켜보자.
혹시,
'The bucket does not allow ACLs (Service: Amazon S3; Status Code: 400; Error Code: AccessControlListNotSupported'
라는 오류가 발생했을 시, https://fun-coding-study.tistory.com/324
The bucket does not allow ACLs
The bucket does not allow ACLs (Service: Amazon S3; Status Code: 400; Error Code: AccessControlListNotSupported 버킷의 ACL(Access Control List) 이 비활성화되어 있었다. ACL 활성화됨을 선택한다.
fun-coding-study.tistory.com
S3 버킷에 가보면

이미지가 잘 업로드 된 것을 확인할 수 있다.
'자바스크립트' 카테고리의 다른 글
자바스크립트) 객체의 복사 (0) | 2023.05.30 |
---|---|
React) 구글 로그인 - @react-oauth/google (0) | 2023.03.30 |
React)Discord 로그인 (with Supabase) (0) | 2023.01.11 |
use-query)useInfiniteQuery를 사용해보자. (0) | 2022.12.29 |
use-query) queryClient.invalidateQueries가 동작하지 않을 때. (0) | 2022.12.28 |